Quarkus Azure Blob Storage Extension
Quarkus Azure Services Extensions are developed and supported by Microsoft as part of their commitment to Open Standard Enterprise Java. For more information, see Jakarta EE on Azure.
Azure Blob Storage is a massively scalable and secure object storage for cloud-native workloads, archives, data lakes, high-performance computing, and machine learning.
This extension allows you to store and retrieve blobs from Azure Blob Storage by injecting a com.azure.storage.blob.BlobServiceClient
or com.azure.storage.blob.BlobServiceAsyncClient
object inside your Quarkus application.
This is a step by step guide on how to use the Quarkus Azure Blob Storage extension. If you’re looking for a complete code sample, you can find it in the Azure Blob Storage sample.
Installation
If you want to use this extension, you need to add the io.quarkiverse.azureservices:quarkus-azure-storage-blob
extension first to your build file.
For instance, with Maven, add the following dependency to your POM file:
<dependency>
<groupId>io.quarkiverse.azureservices</groupId>
<artifactId>quarkus-azure-storage-blob</artifactId>
<version>1.1.2</version>
</dependency>
How to Use It
Once you have added the extension to your project, follow the next steps, so you can inject com.azure.storage.blob.BlobServiceClient
or com.azure.storage.blob.BlobServiceAsyncClient
object in your application to store and read blobs.
Setup your Azure Environment
First thing first.
For this sample to work, you need to have an Azure account as well as Azure CLI installed.
The Azure CLI is available to install in Windows, macOS and GNU/Linux environments.
Checkout the installation guide.
Then, you need an Azure subscription and log into it by using the az login
command.
You can run az version
to find the version and az upgrade
to upgrade to the latest version.
Create an Azure resource group with the az group create command. A resource group is a logical container into which Azure resources are deployed and managed.
az group create \
--name rg-quarkus-azure-storage-blob \
--location eastus
Create a general-purpose storage account with the following command:
az storage account create \
--name stquarkusazurestorageblo \
--resource-group rg-quarkus-azure-storage-blob \
--location eastus \
--sku Standard_ZRS \
--encryption-services blob
If you log into the Azure portal, you can see the resource group and the storage account you created.
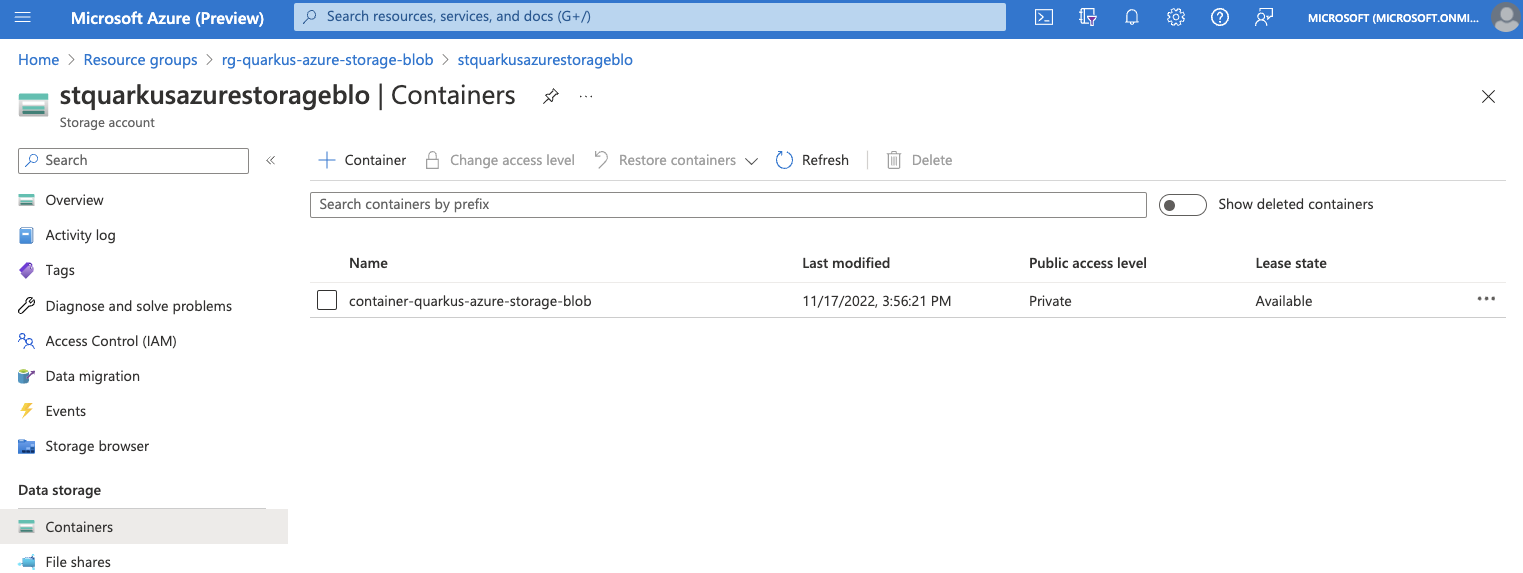
Blobs are always uploaded into a container. You can organize groups of blobs in containers similar to the way you organize your files on your computer in folders. This guide will use the Azure Storage Blob client to create the container if it doesn’t exist. Alternatively, follow instructions in Create a container if you want to create a container before uploading blobs.
Configure the Azure Storage Blob Client
As you can see below in the Configuration Reference section, either the property quarkus.azure.storage.blob.endpoint
or the property quarkus.azure.storage.blob.connection-string
is required if the extension is enabled.
You have two options to authenticate to Azure Storage Blob, either with Microsoft Entra ID or connection string. The following sections describe how to authenticate with both options. For optimal security, it is recommended to use Microsoft Entra ID for authentication.
Authenticating to Azure Storage Blob with Microsoft Entra ID
You can authenticate to Azure Storage Blob with Microsoft Entra ID. Run the following commands to assign the Storage Blob Data Contributor
role to the signed-in user as a Microsoft Entra identity.
# Retrieve the storage account resource ID
STORAGE_ACCOUNT_RESOURCE_ID=$(az storage account show \
--resource-group rg-quarkus-azure-storage-blob \
--name stquarkusazurestorageblo \
--query 'id' \
--output tsv)
# Assign the "Storage Blob Data Contributor" role to the current signed-in identity
az role assignment create \
--assignee $(az ad signed-in-user show --query 'id' --output tsv) \
--role "Storage Blob Data Contributor" \
--scope $STORAGE_ACCOUNT_RESOURCE_ID
Then, export Azure Storage Blob endpoint as an environment variable.
export QUARKUS_AZURE_STORAGE_BLOB_ENDPOINT=$(az storage account show \
--resource-group rg-quarkus-azure-storage-blob \
--name stquarkusazurestorageblo \
--query 'primaryEndpoints.blob' \
--output tsv)
echo "QUARKUS_AZURE_STORAGE_BLOB_ENDPOINT is: ${QUARKUS_AZURE_STORAGE_BLOB_ENDPOINT}"
The value of environment variable QUARKUS_AZURE_STORAGE_BLOB_ENDPOINT
will be read by Quarkus as the value of config
property quarkus.azure.storage.blob.endpoint
in order to set up the connection to the Azure Storage Blob.
Authenticating to Azure Storage Blob with connection string
You can also authenticate to Azure Storage Blob with connection string. Run the following commands to export the Azure Storage Blob connection string as an environment variable.
export QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING=$(az storage account show-connection-string \
--resource-group rg-quarkus-azure-storage-blob \
--name stquarkusazurestorageblo \
--output tsv)
echo "QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING is: ${QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING}"
The value of environment variable QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING
will be fed into config
property quarkus.azure.storage.blob.connection-string
in order to set up the connection to the Azure Storage Blob.
Notice that you get the connection string and set it to environment variable QUARKUS_AZURE_STORAGE_BLOB_CONNECTION_STRING
, instead of setting it to property quarkus.azure.storage.blob.connection-string
in the application.properties
file.
Although technically both approaches work, using environment variable is recommended and more secure as there’s no risk of committing the connection string to source control.
Inject the Azure Storage Blob Client
Now that your Azure environment is ready and that you have configured the extension, you can inject the com.azure.storage.blob.BlobServiceClient
object in your imperative application or inject the com.azure.storage.blob.BlobServiceAsyncClient
object in your reactive application, so you can interact with Azure Blob Storage.
Use the BlobServiceClient in an imperative application
The uploadBlob
method first creates the container container-quarkus-azure-storage-blob
, sets some text to a text file, and then uploads the text to the container.
The downloadBlob
method downloads the text file from the container and prints the text to the console.
@Path("/quarkus-azure-storage-blob")
@ApplicationScoped
public class StorageBlobResource {
@Inject
BlobServiceClient blobServiceClient;
@POST
public Response uploadBlob() {
BlobContainerClient blobContainerClient = blobServiceClient
.createBlobContainerIfNotExists("container-quarkus-azure-storage-blob");
BlobClient blobClient = blobContainerClient.getBlobClient("quarkus-azure-storage-blob.txt");
blobClient.upload(BinaryData.fromString("Hello quarkus-azure-storage-blob at " + LocalDateTime.now()), true);
return Response.status(CREATED).build();
}
@GET
public String downloadBlob() {
BlobContainerClient blobContainerClient = blobServiceClient
.getBlobContainerClient("container-quarkus-azure-storage-blob");
BlobClient blobClient = blobContainerClient.getBlobClient("quarkus-azure-storage-blob.txt");
return blobClient.downloadContent().toString();
}
}
To test this sample you can run the following cURL commands after the application is started:
-
curl -X POST localhost:8080/quarkus-azure-storage-blob
-
curl localhost:8080/quarkus-azure-storage-blob
You can go back to the Azure portal and see the container container-quarkus-azure-storage-blob
and the blob quarkus-azure-storage-blob.txt
that you’ve created.
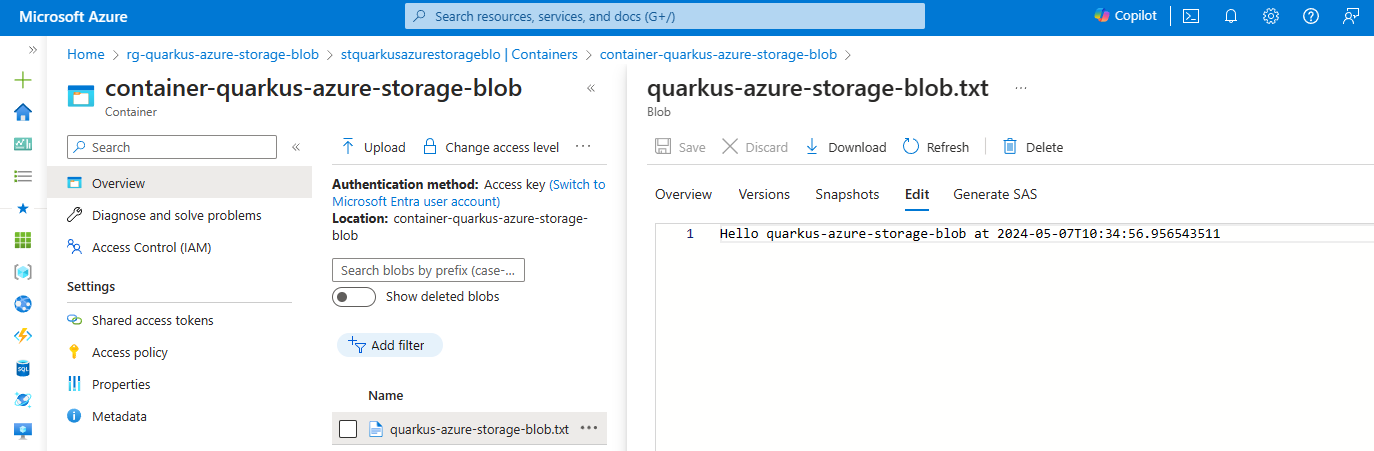
Use the BlobServiceAsyncClient in a reactive application
Similarly, the uploadBlob
method first asynchronously creates the container container-quarkus-azure-storage-blob-async
, sets some text to a text file, and then uploads the text to the container.
The downloadBlob
method asynchronously downloads the text file from the container and prints the text to the console.
@Path("/quarkus-azure-storage-blob-async")
@ApplicationScoped
public class StorageBlobAsyncResource {
@Inject
BlobServiceAsyncClient blobServiceAsyncClient;
@POST
public Uni<Response> uploadBlob() {
Mono<BlockBlobItem> blockBlobItem = blobServiceAsyncClient
.createBlobContainerIfNotExists("container-quarkus-azure-storage-blob-async")
.map(it -> it.getBlobAsyncClient("quarkus-azure-storage-blob-async.txt"))
.flatMap(it -> it.upload(BinaryData.fromString("Hello quarkus-azure-storage-blob-async at " + LocalDateTime.now()), true));
return Uni.createFrom().completionStage(blockBlobItem.toFuture()).map(it -> Response.status(CREATED).build());
}
@GET
public Uni<Response> downloadBlob() {
BlobAsyncClient blobAsyncClient = blobServiceAsyncClient.getBlobContainerAsyncClient("container-quarkus-azure-storage-blob-async")
.getBlobAsyncClient("quarkus-azure-storage-blob-async.txt");
return Uni.createFrom()
.completionStage(blobAsyncClient.downloadContent().map(it -> Response.ok().entity(it.toString()).build())
.toFuture());
}
}
To test this sample you can run the following cURL commands after the application is started:
-
curl -X POST localhost:8080/quarkus-azure-storage-blob-async
-
curl localhost:8080/quarkus-azure-storage-blob-async
You can also go back to the Azure portal and see the container container-quarkus-azure-storage-blob-async
and the blob quarkus-azure-storage-blob-async.txt
that you’ve created.
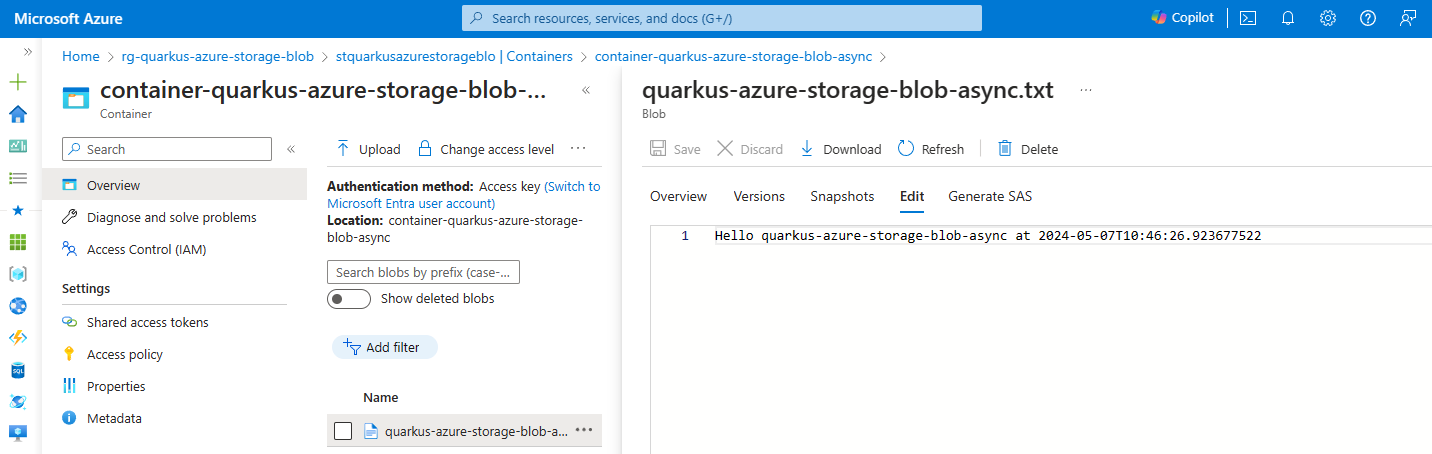
Extension Configuration Reference
Configuration property fixed at build time - All other configuration properties are overridable at runtime
Configuration property |
Type |
Default |
---|---|---|
Whether a health check is published in case the smallrye-health extension is present. Environment variable: |
boolean |
|
If DevServices has been explicitly enabled or disabled. DevServices is generally enabled by default, unless there is an existing configuration present. When DevServices is enabled Quarkus will attempt to automatically configure and start an azurite instance when running in Dev or Test mode and when Docker is running. Environment variable: |
boolean |
|
The container image name to use, for container based DevServices providers. Environment variable: |
string |
|
Optional fixed port the Dev services will listen to. If not defined, the port will be chosen randomly. Environment variable: |
int |
|
Indicates if the azurite instance managed by Quarkus Dev Services is shared. When shared, Quarkus looks for running containers using label-based service discovery. If a matching container is found, it is used, and so a second one is not started. Otherwise, Dev Services for Azure Storage Blob starts a new container. The discovery uses the Container sharing is only used in dev mode. Environment variable: |
boolean |
|
The value of the This property is used when you need multiple shared azurite instances. Environment variable: |
string |
|
The flag to enable the storage blob. If set to false, the storage blob will be disabled Environment variable: |
boolean |
|
The endpoint of Azure Storage Blob. Required if quarkus.azure.storage.blob.enabled is set to true and quarkus.azure.storage.blob.connection-string is not set Environment variable: |
string |
|
The connection string of Azure Storage Blob. Required if quarkus.azure.storage.blob.enabled is set to true and quarkus.azure.storage.blob.endpoint is not set Environment variable: |
string |