Quarkus Azure Key Vault Extension
Quarkus Azure Services Extensions are developed and supported by Microsoft as part of their commitment to Open Standard Enterprise Java. For more information, see Jakarta EE on Azure.
Azure Key Vault is a cloud service for securely storing and accessing secrets. A secret is anything that you want to tightly control access to, such as API keys, passwords, certificates, or cryptographic keys. This extension allows you to:
-
Create and retrieve secrets from Azure Key Vault by injecting the one or both of the following objects in a Quarkus application.
-
com.azure.security.keyvault.secrets.SecretClient
-
com.azure.security.keyvault.secrets.SecretAsyncClient
-
-
Use secrets from the key vault directly inside your
application.properties
.
The extension produces SecretClient
and SecretAsyncClient
using DefaultAzureCredential.
Developers who want more control or whose scenario isn’t served by the default settings should build their clients using other credential types.
Installation
Add the io.quarkiverse.azureservices:quarkus-azure-keyvault
extension first to your build file.
For instance, with Maven, add the following dependency to your POM file:
<dependency>
<groupId>io.quarkiverse.azureservices</groupId>
<artifactId>quarkus-azure-keyvault</artifactId>
<version>1.1.6</version>
</dependency>
How to Use It
Once you have added the extension to your project, follow the next steps, so you can inject com.azure.security.keyvault.secrets.SecretClient
or com.azure.security.keyvault.secrets.SecretAsyncClient
in your application to manage secrets.
Setup your Azure Environment
First thing first.
For this sample to work, you need to have an Azure account as well as Azure CLI installed.
The Azure CLI is available to install in Windows, macOS and GNU/Linux environments.
Checkout the installation guide.
Then, you need an Azure subscription and log into it by using the az login
command.
You can run az version
to find the version and az upgrade
to upgrade to the latest version.
Create an Azure resource group with the az group create
command.
A resource group is a logical container into which Azure resources are deployed and managed.
az group create \
--name rg-quarkus-azure-keyvault \
--location eastus
Create a general-purpose key vault with the following command:
az keyvault create --name kvquarkusazurekv0423 \
--resource-group rg-quarkus-azure-keyvault \
--location eastus \
--enable-rbac-authorization false
Key Vault provides secure storage of generic secrets, such as passwords and database connection strings. All secrets in your key vault are stored encrypted. The Azure Key Vault service encrypts your secrets when you add them, and decrypts them automatically when you read them.
The command creating key vault has assigned all secret permissions(backup, delete, get, list, purge, recover, restore, set) to your signed in identity:
Create a secret secret1 with value mysecret
.
az keyvault secret set \
--vault-name kvquarkusazurekv0423 \
--name secret1 \
--value mysecret
If you sign into the Azure portal, you can see the key vault you created. Select Objects → Secrets, you will find the Secrets page.
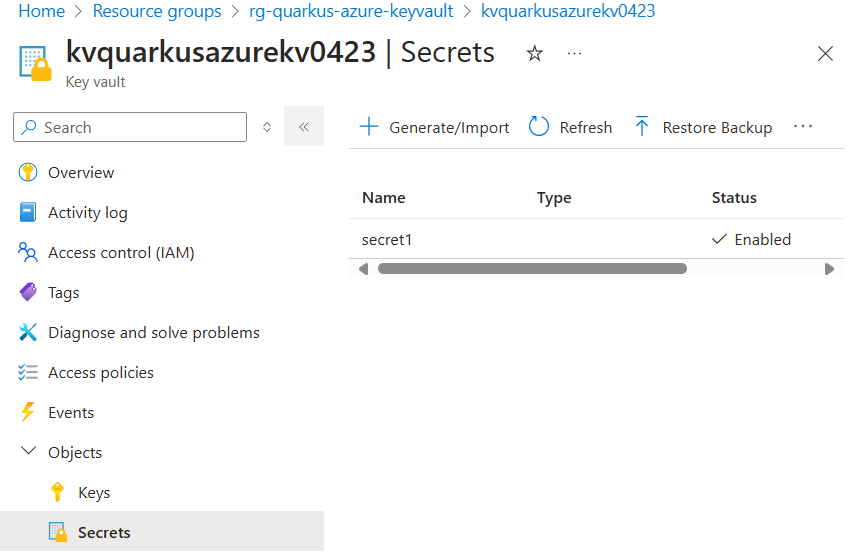
Configure the Azure Key Vault Secret Client
As you can see below in the Configuration Reference section, the configuration option quarkus.azure.keyvault.secret.endpoint
is mandatory.
To get the endpoint, execute the following Azure CLI command:
export QUARKUS_AZURE_KEYVAULT_SECRET_ENDPOINT=$(az keyvault show --name kvquarkusazurekv0423 \
--resource-group rg-quarkus-azure-keyvault \
--query properties.vaultUri \
--output tsv)
Notice that you get the endpoint and set it to environment variable QUARKUS_AZURE_KEYVAULT_SECRET_ENDPOINT
, instead of setting it to property quarkus.azure.keyvault.secret.endpoint
in the application.properties
file.
Due to Quarkus implementing the MicroProfile Config specification, both approaches work. Using the environment variable is recommended, more flexible and more secure as there’s no risk of committing the endpoint to source control.
Read Secrets as Properties
You can load and reference secrets from Azure Key Vault in your application.properties file by using the following syntax. A hierarchical approach is supported, similar to that found in Google Cloud Secret Manager.
The syntax supports loading secrets from multiple key vault. Default key vault is configed with quarkus.azure.keyvault.secret.endpoint
.
# 1. Load secret from default key vault - specify the secret name and use the latest version
${kv//<secret-name>}
# 2. Load secret from default key vault - specify the secret name and version
${kv//<secret-name>/versions/<version-id>}
# 3. Load secret from a specified key vault - specify key vault name, secret name, and use latest version
${kv//<keyvault-name>/<secret-name>}
# 4. Load secret from a specified key vault - specify key vault name, secret name, and use latest version
${kv//<keyvault-name>/secrets/<secret-name>}
# 5. Load secret from a specified key vault - specify key vault name, secret name, and version
${kv//<keyvault-name>/secrets/<secret-name>/<version-id>}
# 6. Load secret from a specified key vault - specify key vault name, secret name, and version
${kv//<keyvault-name>/secret/<secret-name>/versions/<version-id>}
You can use this syntax to load secrets directly from application.properties
:
@ConfigProperty(name = "kv//secret1")
String secret;
Inject the Azure Key Vault Secret Client
Now that your Azure environment is ready and that you have configured the extension, you can inject the com.azure.security.keyvault.secrets.SecretClient
object in your application, so you can interact with Azure Key Vault Secret.
This is a Quarkus CLI application. The application will:
-
Ask for a secret value.
-
Create a secret with name
mySecret
and set its value. -
Retrieve and print the secret value.
-
Delete the secret.
You can build and run the application in development mode using command:
quarkus dev
@QuarkusMain
public class KeyVaultSecretApplication implements QuarkusApplication {
@Inject
SecretClient secretClient;
@ConfigProperty(name = "kv//secret1")
String secret;
@Override
public int run(String... args) throws Exception {
System.out.println("Print secret1: " + secret);
Console con = System.console();
String secretName = "mySecret";
System.out.println("Create secret: " + secretName);
System.out.println("Please provide the value of your secret > ");
String secretValue = con.readLine();
System.out.println("Creating a secret called '" + secretName + "' with value '" + secretValue + "' ... ");
secretClient.setSecret(new KeyVaultSecret(secretName, secretValue));
System.out.println("Retrieving your secret...");
KeyVaultSecret retrievedSecret = secretClient.getSecret(secretName);
System.out.println("Your secret's value is '" + retrievedSecret.getValue() + "'.");
System.out.println("Deleting your secret ... ");
SyncPoller<DeletedSecret, Void> deletionPoller = secretClient.beginDeleteSecret(secretName);
deletionPoller.waitForCompletion();
System.out.println("done.");
return 0;
}
}
After running the application, if you log into the Azure portal, you can see the key vault and the secret you created. As the secret is deleted, you will find the secret from Objects → Secrets → Manage deleted secrets.
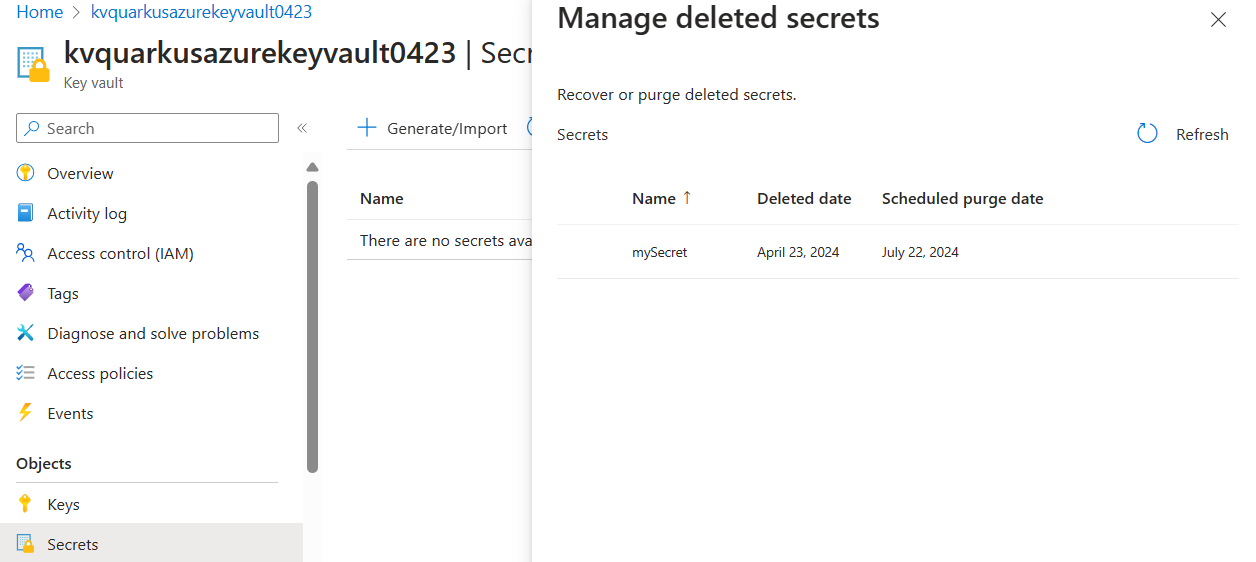
You can also inject com.azure.security.keyvault.secrets.SecretAsyncClient
object to your application. For more usage, see com.azure.security.keyvault.secrets.secretasyncclient.
After the testing, for security consideration, you can rotate the policy with command:
az ad signed-in-user show --query id -o tsv \
| az keyvault delete-policy \
--name kvquarkusazurekv0423 \
--object-id @-
Extension Configuration Reference
Configuration property fixed at build time - All other configuration properties are overridable at runtime
Configuration property |
Type |
Default |
---|---|---|
The flag to enable the key vault secret. If set to false, the key vault secret will be disabled Environment variable: |
boolean |
|
The endpoint of Azure Key Vault Secret. Required if quarkus.azure.keyvault.secret.enabled is set to true Environment variable: |
string |