Quarkus Cucumber
This extension allows you to use Cucumber to test your Quarkus application.
Installation
If you want to use this extension, you need to add the io.quarkiverse.cucumber:quarkus-cucumber
extension first.
In your pom.xml
file, add:
<dependency>
<groupId>io.quarkiverse.cucumber</groupId>
<artifactId>quarkus-cucumber</artifactId>
<version>1.3.0</version>
</dependency>
Usage
To bootstrap Cucumber add the following class to your test suite:
import io.quarkiverse.cucumber.CucumberQuarkusTest;
public class MyTest extends CucumberQuarkusTest {
}
This will automatically bootstrap Cucumber, and discover any .feature
files and step classes that provide glue code.
ScenarioScope
The @ScenarioScope
annotation allows you to define beans whose state is tied to the lifecycle of a Cucumber scenario. This means that the state of these beans will automatically reset between the execution of each scenario, without the need for manual cleanup.
This feature is particularly useful for managing stateful beans in Cucumber tests, similar to the mechanism provided by Spring, as described in the Cucumber documentation.
Example
The usage of @ScenarioScope
is similar to other CDI scopes, such as @ApplicationScoped
.
Here’s how you can define a @ScenarioScope
bean and use it within your step definitions:
import io.quarkiverse.cucumber.ScenarioScope;
import jakarta.inject.Inject;
@ScenarioScope
public class MyStatefulBean {
private String state;
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
}
public class MyStepDefinitions {
@Inject
MyStatefulBean myStatefulBean;
@Given("I set the state to {string}")
public void setState(String state) {
myStatefulBean.setState(state);
}
}
In this example, MyStatefulBean
is injected into the step definition class, and each scenario will have its own instance of the bean. This ensures that the state is isolated across different scenarios.
IDE Integration
The test class can by run by any IDE with support for JUnit5.
In IntelliJ it is possible to directly run feature files:
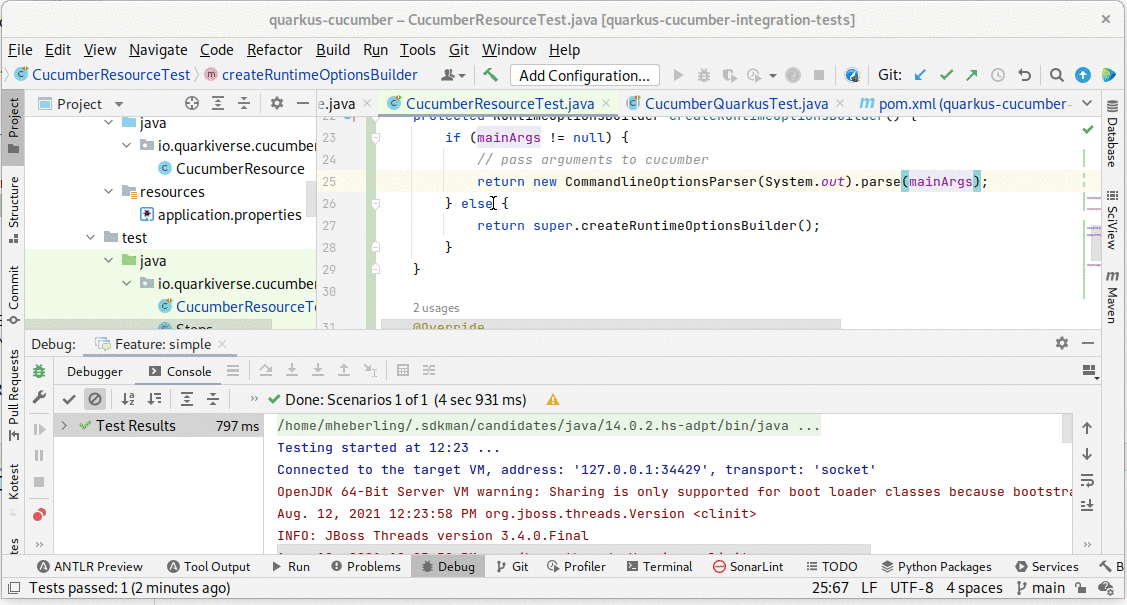
You need to add the following main
method to your test class:
import io.quarkiverse.cucumber.CucumberQuarkusTest;
public class MyTest extends CucumberQuarkusTest {
public static void main(String[] args) {
runMain(MyTest.class, args);
}
}